목차
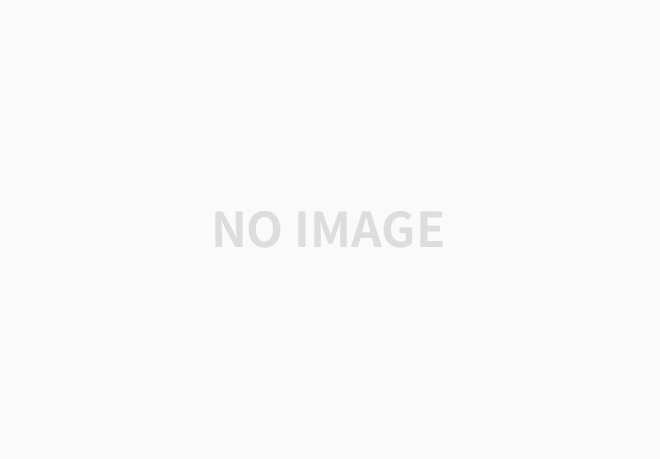
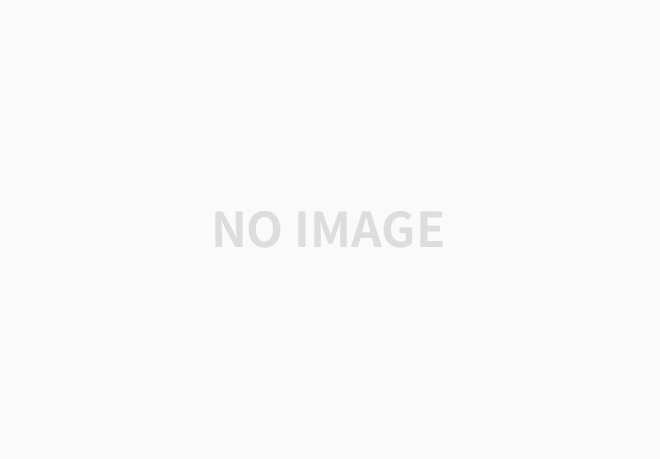
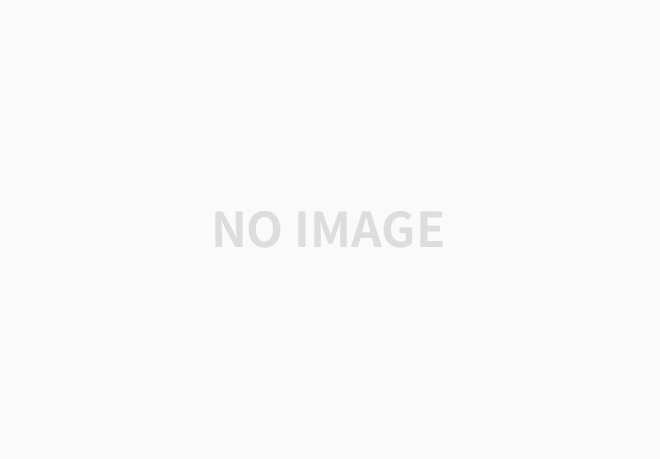
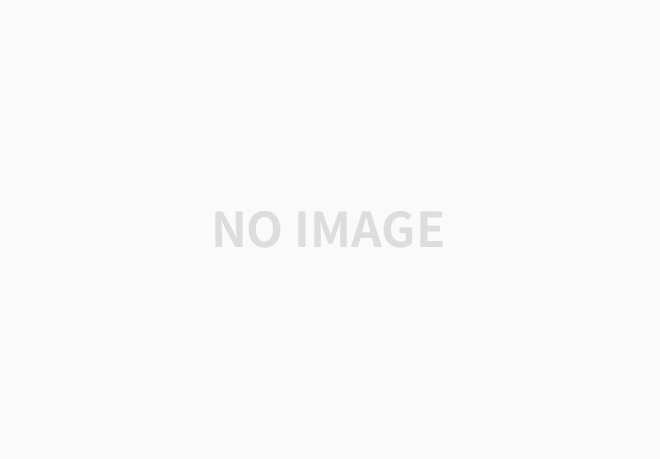
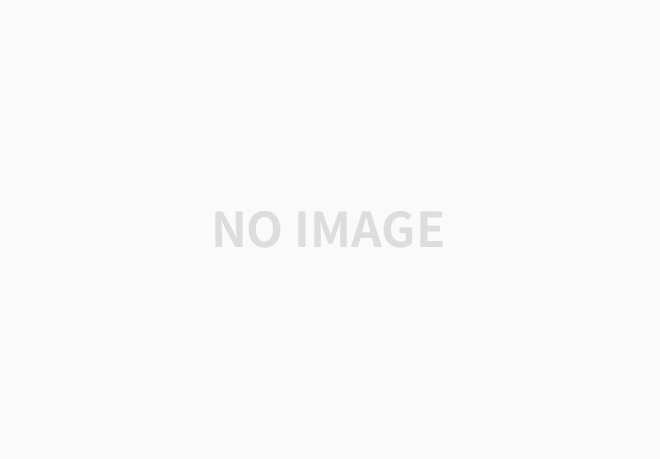
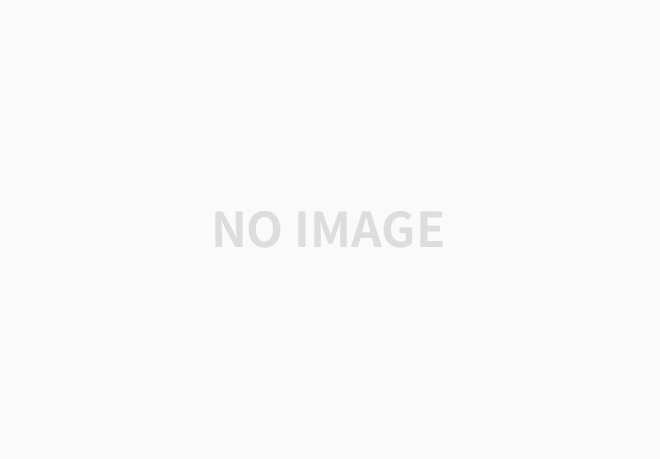
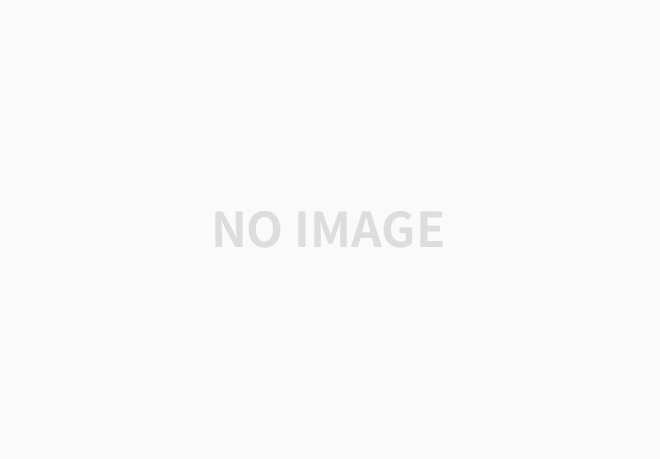
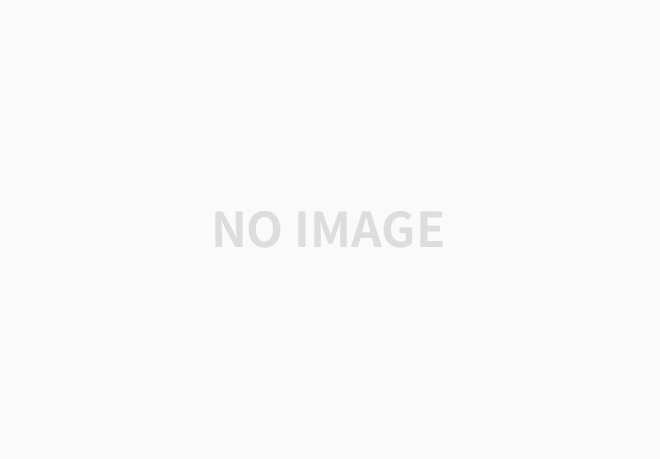
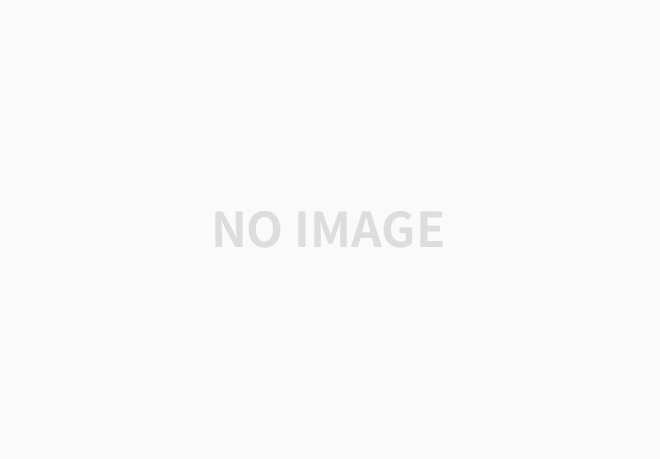
root/
settings.py
ALLOWED_HOSTS = ["*"]
# Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'home',
'dept',
'emp',
'accounts',
]
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [BASE_DIR / 'templates'],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': BASE_DIR / 'db.sqlite3',
}
}
urls.py
from django.contrib import admin
from django.urls import path, include
import dept.views
import emp.views
import home.views
urlpatterns = [
path('',home.views.index),
path('intro',home.views.intro),
path('dept/',dept.views.list, name='deptList'),
path('dept/add',dept.views.add),
path('dept/<int:deptno>',dept.views.detail, name='deptDetail'),
path('emp/',emp.views.list),
path('admin/', admin.site.urls),
path("accounts/", include("accounts.urls")),
]
dept/
admin.py
from django.contrib import admin
from dept.models import Dept
# Register your models here.
admin.site.register(Dept)
views.py
from django.http import HttpResponse, JsonResponse
from django.shortcuts import render, redirect
from dept.models import Dept
import json
# Create your views here.
def list(req):
# return HttpResponse('dept test page')
result=Dept.objects.all()
context={'result':result}
return render(req,'dept/index.html',context)
def add(req):
# print(req.method)
# for msg in dir(req):
# if not msg.startswith('_'): print(msg)
if req.method=='GET':
return render(req,'dept/add.html')
elif req.method=='POST':
dname,loc=req.POST['dname'],req.POST['loc']
dept=Dept(dname=dname,loc=loc)
dept.save()
return redirect('deptList')
else:
return HttpResponse('test ok')
def detail(req,deptno):
print(req.method)
# print(1,Dept.objects.all()[0])
# print(2,Dept.objects.filter(deptno=deptno)[0])
# print(3,Dept.objects.get(deptno=deptno))
# print(4,Dept.objects.filter(dname__contains='test'))
# print(5,Dept.objects.filter(deptno__gt=1))
# print(6,Dept.objects.filter(deptno__lt=3))
# dept=Dept.objects.filter(deptno=deptno)[0] if len(Dept.objects.filter(deptno=deptno))>0 else None
# context={'dept':dept}
# return render(req, 'dept/detail.html', context)
if req.method=='GET':
try:
dept = Dept.objects.get(deptno=deptno)
context = {'dept': dept}
return render(req,'dept/detail.html',context)
except:
return render(req, 'dept/detail.html', {'dept':None})
elif req.method=='PUT':
try:
paramJson=json.loads(req.body)
dept=Dept.objects.get(deptno=deptno)
dept.dname=paramJson['dname']
dept.loc=paramJson['loc']
dept.save()
return JsonResponse(data={"result":"success"})
except Exception as e:
# return JsonResponse(data={"result":"error","err":e})
raise e
elif req.method=='DELETE':
Dept.objects.get(deptno=deptno).delete()
return JsonResponse(data={"result":"success"})
models.py
from django.db import models
# Create your models here.
class Dept(models.Model):
deptno=models.IntegerField(auto_created=True,primary_key=True,serialize=True)
dname=models.CharField(max_length=10)
loc=models.TextField()
def __str__(self):
return '{:0>4s}.'.format(str(self.deptno))+self.dname
accounts/
views.py
from django.shortcuts import render, redirect
# from django.contrib.auth.models import User'
from accounts.models import User
# from django.contrib.auth import login, logout, authenticate
# from django.contrib.auth.decorators import login_required
from django.http import HttpResponse
def user_signup(request):
if request.method == "POST":
username = request.POST["username"]
password = request.POST["password"]
email = request.POST.get("email", "")
if not (username and password):
return HttpResponse("이름과 패스워드는 필수입니다.")
if User.objects.filter(username=username).exists():
return HttpResponse("유저이름이 이미 있습니다.")
if email and User.objects.filter(email=email).exists():
return HttpResponse("이메일이 이미 있습니다.")
# user = User.objects.create_user(username, email, password)
# user.save()
# user = authenticate(username=username, password=password)
user=User(username=username,email=email,password=password)
user.save()
# login(request, user)
return redirect("user_login")
else:
return render(request, "accounts/signup.html")
def user_login(request):
if request.method == "POST":
username = request.POST["username"]
password = request.POST["password"]
# user = authenticate(request, username=username, password=password)
user=User.objects.filter(username=username,password=password)
if user is not None:
# login(request, user)
request.session['username']=username
# request.session['email']=user.email
return redirect("user_profile")
else:
return render(
request,
"accounts/login.html",
{"error": "아이디나 패스워드가 맞지 않습니다."},
)
else:
return render(request, "accounts/login.html")
def user_logout(request):
# logout(request)
print(request.session)
request.session.clear()
return redirect("user_login")
# @login_required
def user_profile(request):
sessionUser=request.session.get('username')
if sessionUser != None:
return render(request, "accounts/profile.html", {"user": sessionUser})
else:
return redirect('user_login')
models.py
from django.db import models
# Create your models here.
class User(models.Model):
username=models.CharField(max_length=5)
email=models.CharField(max_length=20,db_index=True)
password=models.IntegerField(max_length=16)
urls.py
from django.urls import path
from . import views
urlpatterns = [
path("signup/", views.user_signup, name="user_signup"),
path("login/", views.user_login, name="user_login"),
path("logout/", views.user_logout, name="user_logout"),
path("profile/", views.user_profile, name="user_profile"),
]
templates
base/template.html
<!DOCTYPE html>
{% load static %}
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" type="text/css" href="{% static '/css/bootstrap.min.css' %}">
</head>
<body>
<nav class="navbar navbar-default">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="/">
인제대학교
</a>
</div>
<div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1">
<ul class="nav navbar-nav">
<li><a href="/">home</a></li>
<li><a href="/intro">intro</a></li>
<li><a href="/dept/">dept</a></li>
<li><a href="/emp/">emp</a></li>
<li><a href="/accounts/login/">login</a></li>
<li><a href="/accounts/signup/">signup</a></li>
</ul>
</div>
</div>
</nav>
<div class="container">
{% block content %}
{% endblock %}
<footer>
<address>
김해캠퍼스 (50834) 경남 김해시 인제로 197TEL. 055-334-7111<br/>
Copyright(c) 1996-2022 INJE University. All rights reserved.
</address>
</footer>
</div>
</body>
</html>
dept
add
{% extends 'base/template.html' %}
{% block content %}
<h2>Add page(DEPT)</h2>
<form method="post">
{% csrf_token %}
<div class="form-group">
<input name="dname" placeholder="dname" class="form-control" value=""/>
</div>
<div class="form-group">
<input name="loc" placeholder="loc" class="form-control" value=""/>
</div>
<div class="form-group">
<button class="btn btn-primary btn-block">입력</button>
<button type="reset" class="btn btn-default btn-block">취소</button>
<button type="button" class="btn btn-default btn-block" onclick="history.back();">뒤로</button>
</div>
</form>
{% endblock %}
detail
{% extends 'base/template.html' %}
{% block content %}
<h2>Detail page(DEPT)</h2>
<form method="post">
{% csrf_token %}
<div class="form-group">
<input name="deptno" placeholder="deptno" class="form-control" value="{{dept.deptno}}" readonly/>
</div>
<div class="form-group">
<input name="dname" placeholder="dname" class="form-control" value="{{dept.dname}}"/>
</div>
<div class="form-group">
<input name="loc" placeholder="loc" class="form-control" value="{{dept.loc}}"/>
</div>
<div class="form-group">
<button class="btn btn-primary btn-block">수정</button>
<button type="button" class="btn btn-danger btn-block">삭제</button>
<button type="button" class="btn btn-default btn-block" onclick="history.back();">뒤로</button>
</div>
</form>
<script>
const form=document.querySelector('form');
form.querySelectorAll('button')[1].onclick=e=>{
fetch('/dept/{{dept.deptno}}',{
method:'DELETE'
}).then(e=>e.ok).finally(()=>location.href='/dept/')
};
form.onsubmit=e=>{
const dname=form.querySelectorAll('input')[2].value;
const loc=form.querySelectorAll('input')[3].value;
e.preventDefault();
fetch('/dept/{{dept.deptno}}',{
method:'PUT',
body:'{"dname":"'+dname+'","loc":"'+loc+'"}'
})
.then(e=>e.json())
.then(e=>location.href='/dept/')
.catch(err=>{
alert(err);
location.href='/dept/';
})
}
</script>
{% endblock %}
index
{% extends 'base/template.html' %}
{% block content %}
<h2>List page(DEPT)</h2>
<table class="table">
<thead>
<tr>
<th>deptno</th>
<th>dname</th>
<th>loc</th>
</tr>
</thead>
<tbody>
{% for row in result %}
<tr>
<td><a href="{{row.deptno}}">{{row.deptno}}</a></td>
<td><a href="{% url 'deptDetail' row.deptno %}">{{row.dname}}</a></td>
<td><a href="{% url 'deptDetail' row.deptno %}">{{row.loc}}</a></td>
</tr>
{% endfor %}
</tbody>
</table>
<a href="add" class="btn btn-primary btn-block" role="button">입력</a>
{% endblock %}
accounts
login
{% extends 'base/template.html'%}
{% block content %}
<h2>login page</h2>
<form method="post">
{% csrf_token %}
<label for="username_id">아이디</label>
<input id="username_id" type="text" name="username">
<label for="password_id">비밀번호</label>
<input id="password_id" type="password" name="password">
<button type="submit">로그인</button>
</form>
{% endblock %}
profile
{% extends 'base/template.html'%}
{% block content %}
<h1>개인 프로필 페이지</h1>
<p>{{ user }}</p>
<p>{{ user.username }}</p>
<p>{{ user.email }}</p>
<p>{{ user.first_name }}</p>
<p>{{ user.last_name }}</p>
<p>{{ user.is_staff }}</p>
<p>{{ user.is_active }}</p>
<p>{{ user.is_superuser }}</p>
<p>{{ user.last_login }}</p>
<p>{{ user.date_joined }}</p>
<form action="{% url 'user_logout' %}" method="post">
{% csrf_token %}
<input type="submit" value="로그아웃">
</form>
{% endblock %}
signup
{% extends 'base/template.html'%}
{% block content %}
<h2>signup page</h2>
<form action="" method="post">
{% csrf_token %}
<label for="username_id">아이디</label>
<input id="username_id" type="text" name="username">
<label for="email_id">이메일</label>
<input id="email_id" type="text" name="email">
<label for="password_id">비밀번호</label>
<input id="password_id" type="password" name="password">
<button type="submit">회원가입</button>
</form>
{% endblock %}

'100일 챌린지 > 빅데이터기반 인공지능 융합 서비스 개발자' 카테고리의 다른 글
Day 87 - fastAPI 사용하기 (2) (1) | 2024.12.02 |
---|---|
Day 86 - fastAPI 사용하기 (1) (1) | 2024.11.29 |
Day 85 - Django migrate (0) | 2024.11.28 |
Day 85 - Django로 CRUD 프로그램 만들기 (2) function 활용하기 (1) | 2024.11.28 |
Day 85 - Django 사용하기 (0) | 2024.11.28 |