내부 클래스
- 클래스 내부에 존재하는 클래스를 내부 클래스라 부른다.
- jdk 1.4 이전에는 상속 대신에 내부 클래스를 많이 썼다.
- 최근 국내에서는 절차지향적인 코딩이라는 인식이 있어 선호하지 않는 편이다.
- 내부 클래스를 만들면
외부클래스$내부클래스.class
로 컴파일된다.- anonymous를 사용할때 잘 활용된다.
- 안드로이드는 모든것이 내부 클래스로 이루어져 있다.
- 다만 클래스 소스를 찾기 힘들어 국내 협업에서는 잘 사용하지 않는다.
내부 클래스의 4가지 형식
1. Static 클래스 (static inner class)
class Outter02{
static int su1 = 1111;
int su2 = 2222;
// 생성자
public Outter02() {
}
static void func01() {
// 내부 static 불러오기
System.out.println(Inner02.su3);
Inner02.func03();
// 내부 non-static 불러오기
Inner02 inner = new Inner02();
System.out.println(inner.su4);
inner.func04();
}
void func02() {
// 내부 static 불러오기
System.out.println(Inner02.su3);
Inner02.func03();
// 내부 non-static 불러오기
Inner02 inner = new Inner02();
System.out.println(inner.su4);
inner.func04();
}
// static 내부 클래스
static class Inner02{
static int su3 = 3333;
int su4 = 4444;
// 생성자
public Inner02() {
}
static void func03() {
System.out.println(su3);
System.out.println(Outter02.su1);
System.out.println(su1); // Outter에 있는 필드 접근 가능
Outter02.func01(); // 외부 클래스에 있는 메소드 호출 가능
func01(); // 외부 클래스명은 생략가능하다.
Outter02 outt = new Outter02();
System.out.println(outt.su2);
outt.func02(); // 외부 클래스에 있는 non-static 메소드 접근 가능
}
void func04() {
System.out.println(su3);
System.out.println(Outter02.su1);
System.out.println(su1); // Outter에 있는 필드 접근 가능
Outter02.func01(); // 외부 클래스에 있는 메소드 호출 가능
func01(); // 외부 클래스명은 생략가능하다.
Outter02 outt = new Outter02();
System.out.println(outt.su2);
outt.func02(); // 외부 클래스에 있는 non-static 메소드 접근 가능
}
}
}
public class Ex02 {
public static void main(String[] args) {
// 외부 클래스 불러오기
System.out.println(Outter02.su1);
Outter02 outt = new Outter02();
System.out.println(outt.su2);
// 내부 클래스 불러오기
// 1. static 내부 클래스
System.out.println(Outter02.Inner02.su3);
// 2. non-static 내부 클래스
Outter02.Inner02 inn = new Outter02.Inner02();
System.out.println(inn.su4);
}
}
java.util.Map.Entry entry; // 내부 클래스의 활용 예시
2. Instance 클래스 (non-static inner class)
class Outter03{
static int su1 = 1111;
int su2 = 2222;
public Outter03() {
}
static void func01() {
System.out.println(Inner03.su3);
Outter03 outt = new Outter03();
Inner03 inn = outt.new Inner03(); // Outter를 생성하기 전까지는 내부 static 클래스를 생성할 수 없다.
System.out.println(inn.su4);
inn.func04();
}
void func02() {
System.out.println(Inner03.su3);
Inner03 inn = this.new Inner03();
// Inner03 inn = new Inner03();
System.out.println(inn.su4);
inn.func04();
}
// non-static 내부 클래스
class Inner03{
// static int su3 = 3333; // non-static에서는 static 필드를 생성할 수 없다.
final static int su3 = 3333; // 상수형 변수는 메모리에 상수영역이 따로 존재하기 때문에 생성될 수 있다.
int su4 = 4444;
// static void func03() {} // 마찬가지로 존재할 수 없다.
void func04() {
System.out.println(su1);
System.out.println(su2);
func01();
Outter03.func01();
func02();
}
}
}
public class Ex03 {
public static void main(String[] args) {
System.out.println(Outter03.Inner03.su3);
System.out.println(com.Day12.Outter03.Inner03.su3);
Outter03 outt = new Outter03();
Outter03.Inner03 inn = outt.new Inner03();
System.out.println(inn.su4);
inn.func04();
}
}
3. Local 클래스 (지역 클래스)
public class Ex04 {
static int su3 = 3333;
int su4 = 4444;
public static void func01() {
Ex04 me = new Ex04();
int su5 = 5555; // 상수형 변수라는 전재하에 사용된다.
// jdk 1.7 이하에서는 final 표기 생략 불가
// jdk 1.8 이상에서는 final 표기 생략 가능
// static class Local01{} // local 클래스는 static이 존재할 수 없음
class Local01{
final static int su1 = 1111; // 상수형 변수만 static이 존재할 수 있다.
int su2 = 2222;
public Local01() {
}
void func01() {
System.out.println(su1);
System.out.println(su3);
System.out.println(me.su4);
System.out.println(su5);
}
void func02() {
System.out.println(su2);
}
}
// su5++; // 문법상으로 맞지 않아서 사용할 수 없다. su5를 final이라고 생각하면 된다.
Local01 local = new Local01();
System.out.println(Local01.su1);
System.out.println(local.su2);
local.func01();
local.func02();
}
public void func02() {
class Local01{
final static int su1 = 1111; // 상수형 변수만 static이 존재할 수 있다.
int su2 = 2222;
public Local01() {
}
void func01() {
System.out.println(su1);
}
void func02() {
System.out.println(su2);
}
}
Local01 local = new Local01();
System.out.println(Local01.su1);
System.out.println(local.su2);
local.func01();
local.func02();
}
public static void main(String[] args) {
func01();
System.out.println("----");
Ex04 me = new Ex04();
me.func02();
}
}
4. Anonymous 클래스 (익명 클래스)
- 한번만 호출하면 되는 클래스를 위해
class Anony{
void func(){
}
}
public class Ex05 {
public static void func01() {
Anony obj = new Anony(){
void func() {
System.out.println("실행");
}
};
obj.func();
}
public static void main(String[] args) {
func01();
}
}
interface Inter{
void func();
}
public class Ex05 {
int su1 = 1111;
public static Inter func01() {
return new Inter(){ // class Local implements Inter의 클래스명을 지운 익명클래스이다.
int su2 = 2222;
public void func() {
System.out.println("실행");
System.out.println(this.su2);
}
};
}
public static void main(String[] args) {
Inter local = func01();
local.func();
}
}
interface Inter{
void func();
}
public class Ex05 {
int su = 1111;
static Inter local = new Inter(){
public void func() {
System.out.println("실행");
}
};
public static void main(String[] args) {
local.func();
}
}
interface Inter{
void func();
}
public class Ex05 {
public static void main(String[] args) {
Inter local = new Inter(){
public void func() {
System.out.println("실행");
}
};
local.func();
}
}
람다식을 이용한 Anonymous class
interface Inter{
void func();
}
public class Ex05 {
public static void main(String[] args) {
// Lambda
Inter local2 = () -> System.out.println("람다식");
local2.func();
}
}
Anonymous를 이용한 list sorting
public static void main(String[] args) {
List<Integer> list = new ArrayList<Integer>();
list.add(2222);
list.add(4444);
list.add(1111);
list.add(5555);
list.add(3333);
list.sort(new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
// TODO Auto-generated method stub
return o2.intValue() - o1.intValue();
}
});
System.out.println(list);
}
Comparator desc = new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o2.intValue() - o1.intValue();
}
};
Comparator asc = new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o1.intValue() - o2.intValue();
}
};
List<Integer> list = new ArrayList<Integer>();
list.add(2222);
list.add(4444);
list.add(1111);
list.add(5555);
list.add(3333);
list.sort(asc); // 오름차순으로 정렬
System.out.println(list);
list.sort(desc);
System.out.println(list); // 내림차순으로 정렬
GUI
awt(jdk 1.0)으로 나뉜다.
- awt : os에 요청
- 빠르고, 자원 소모가 적다.
- 운영체제에 종속된다.
- swing : java가 직접 drawing 한다.
- awt가 하지 못하는 기능들을 수행 가능
- 좀 더 세련된 인터페이스 (롱혼 테마)
- awt : os에 요청
)와 swing(jdk 1.2또 웹에서 사용하는 css가 있다.
한글이 나오지 않을 때
배치 레이아웃
- 상대속성과 절대속성(absolute)이 존재한다.
- 상대속성은 배치관리자가 알아서 관리하고 바꿀 수 없다.
Flow Layout
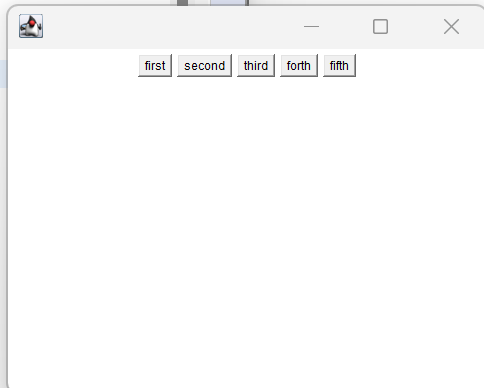
- 가운데 정렬
- 컴포넌트의 배치와 크기를 조절할 수 없다.
- 컨텐츠의 특성(글자의 길이와 크기 등)에 맞춰 사이즈가 조절된다.
public class EX07 extends java.awt.Frame{
public EX07() {
Font f = new Font("궁서체", 1, 20);
// 배치 관리자
LayoutManager l1 = new FlowLayout();
LayoutManager l2 = new GridLayout(3,3);
LayoutManager l3 = new CardLayout();
this.setLayout(l1);
// 버튼 만들기
java.awt.Button btn = new Button();
// btn.setFont(f);
btn.setLabel("first");
this.add(btn);
java.awt.Button btn2 = new Button();
btn2.setLabel("second");
this.add(btn2);
java.awt.Button btn3 = new Button();
btn3.setLabel("third");
this.add(btn3);
java.awt.Button btn4 = new Button();
btn4.setLabel("forth");
this.add(btn4);
java.awt.Button btn5 = new Button();
btn5.setLabel("fifth");
this.add(btn5);
// 초기 창 위치 지정하기
this.setLocation(2560/2-250, 1600/2-200);
// 초기 창 크기 정하기
this.setSize(500, 400);
this.setVisible(true);
}
public static void main(String[] args) {
EX07 me = new EX07();
}
}
Grid Layout
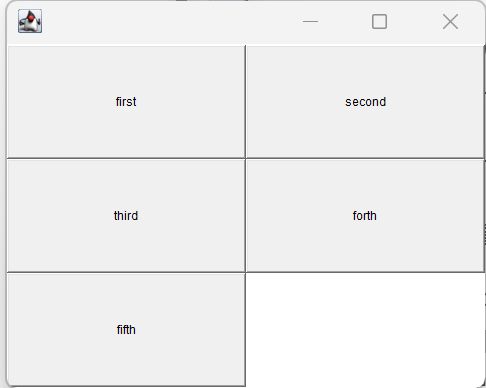
LayoutManager l2 = new GridLayout(3, 2);
- row가 column보다 우선순위를 받는다.
Border Layout
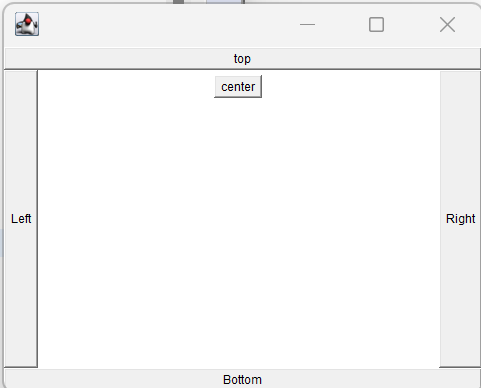
public class Ex08 {
public static void main(String[] args) {
Frame f = new Frame();
LayoutManager lm;
lm = new BorderLayout();
f.setLayout(lm);
Button btn1 = new Button();
btn1.setLabel("top");
f.add(btn1, BorderLayout.NORTH);
Panel center = new Panel();
Button btn2 = new Button();
btn2.setLabel("center");
center.add(btn2);
f.add(center, "Center");
Button btn3 = new Button();
btn3.setLabel("Left");
f.add(btn3, "West");
Button btn4 = new Button();
btn4.setLabel("Right");
f.add(btn4, "East");
Button btn5 = new Button();
btn5.setLabel("Bottom");
f.add(btn5, "South");
f.setLocation(2560/2-250, 1600/2-200);
f.setSize(500,400);
f.setVisible(true);
}
}
Card Layout
public class Ex09 extends Frame{
CardLayout lm = new CardLayout();
public Ex09() {
this.setLayout(lm);
Panel p1 = new Panel();
Panel p2 = new Panel();
Panel p3 = new Panel();
Panel p4 = new Panel();
Color c1 = new Color(255, 0, 0);
p1.setBackground(c1);
Color c2 = new Color(0, 255, 0);
p2.setBackground(c2);
Color c3 = new Color(0, 0, 255);
p3.setBackground(c3);
Color c4 = new Color(200, 0, 200);
p4.setBackground(c4);
add(p1);
add(p2);
add(p3);
add(p4);
setSize(300, 600);
setLocation(2560/2-250, 1600/2-200);
setVisible(true);
}
public static void main(String[] args) {
Ex09 me = new Ex09();
java.util.Scanner sc = new java.util.Scanner(System.in);
while(true) {
int su = sc.nextInt();
if(su == 0) break;
me.lm.next(me);
}
}
}
GridBag Layout
public class Ex10 extends Frame {
public Ex10() {
LayoutManager lm = new GridBagLayout();
setLayout(lm);
Button btn1 = new Button();
Button btn2 = new Button();
Button btn3 = new Button();
Button btn4 = new Button();
Button btn5 = new Button();
Button btn6 = new Button();
btn1.setLabel("btn1");
btn2.setLabel("btn2");
btn3.setLabel("btn3");
btn4.setLabel("btn4");
btn5.setLabel("btn5");
btn6.setLabel("btn6");
GridBagConstraints bag;
bag = new GridBagConstraints();
bag.fill = 0;
bag.gridwidth = 1;
bag.gridheight = 1;
bag.weightx = 1.0;
bag.weighty = 1.0;
bag.gridx = 0;
bag.gridy = 0;
add(btn1, bag);
bag.gridx = 1;
add(btn2, bag);
bag.gridx = 2;
add(btn3, bag);
bag.gridx = 0;
bag.gridy = 1;
add(btn4, bag);
bag.gridx = 1;
add(btn5, bag);
bag.gridx = 2;
add(btn6, bag);
setSize(500, 400);
setLocation(2560/2-250, 1600/2-200);
setVisible(true);
}
public static void main(String[] args) {
new Ex10();
}
}
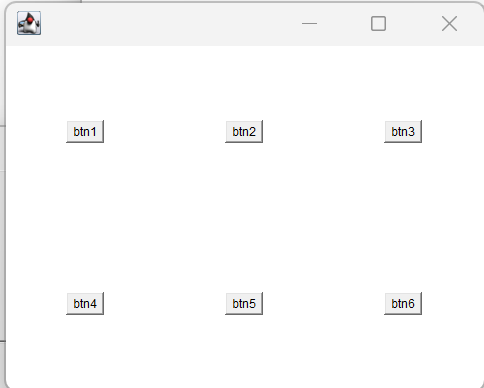
bag.fill = GridBagConstraints.CENTER;
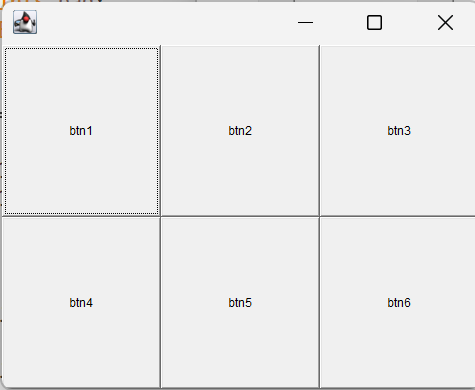
bag.fill = GridBagConstraints.BOTH;
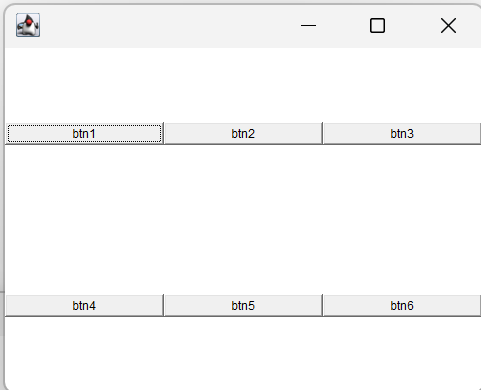
bag.fill = GridBagConstraints.HORIZONTAL;
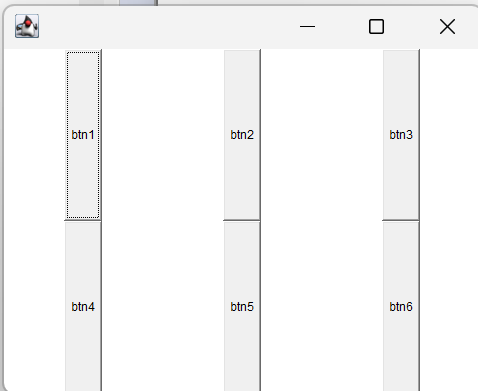
bag.fill = GridBagConstraints.VERTICAL;
Absolute Layout
public class Ex11 extends Frame{
public Ex11() {
setLayout(null); // 절대 관리자
Button btn = new Button();
btn.setLabel("button");
btn.setLocation(200, 200);
btn.setSize(100, 30);
add(btn);
setSize(500, 400);
setResizable(false);
setLocation(2560/2-250, 1600/2-200);
setVisible(true);
}
public static void main(String[] args) {
new Ex11();
}
}
Components
public static void main(String[] args) {
Frame f = new Frame();
Panel p = new Panel();
// p.setLayout(new FlowLayout); // default layout
// p.setBackground(new Color(255, 0, 0));
p.setBackground(Color.PINK);
Button btn = new Button("press");
Font font = Font.getFont(Font.SANS_SERIF);
btn.setFont(font);
btn.setBackground(Color.BLUE);
btn.setForeground(Color.WHITE);
p.add(btn);
JButton btn2 = new JButton("스윙버튼");
btn2.setFont(Font.getFont("궁서체"));
Icon defaultIcon = new ImageIcon("logo.jpg");
btn2.setIcon(defaultIcon);
p.add(btn2);
f.add(p);
f.setTitle("제목없음");
f.setSize(500, 400);
f.setLocation(2560/2-250, 1600/2-200);
f.setVisible(true);
// swing으로 띄우기
// JFrame f = new JFrame();
// f.setSize(300, 300);
// f.setLocation(1500, 1000);
// f.setVisible(true);
}
}
TextField
public class Ex13 extends Frame{
static TextField tf;
public Ex13() {
setLayout(new FlowLayout());
// TextField tf = new TextField("글자", 15);
tf = new TextField("글자", 15);
Font f = new Font("궁서체", 1, 20);
tf.setFont(f);
// tf.setText("입력해주세요");
add(tf);
setSize(500, 400);
setLocation(2560/2-250, 1600/2-200);
setVisible(true);
}
public static void main(String[] args) {
new Ex13();
String msg = tf.getText();
System.out.println(msg);
}
}
TextArea
public class Ex14 {
public static void main(String[] args) {
Frame f = new Frame();
f.setLayout(new FlowLayout());
// TextArea ta = new TextArea("초기값", 5, 15, 3);
TextArea ta = new TextArea("초기값", 5, 15, TextArea.SCROLLBARS_VERTICAL_ONLY);
// ta.setText("abc \nABC");
ta.setFont(new Font("", Font.ITALIC, 30));
ta.setBackground(Color.GRAY);
ta.setForeground(Color.CYAN);
f.add(ta);
f.setSize(500, 400);
f.setLocation(2560/2-250, 1600/2-200);
f.setVisible(true);
}
}
Checkbox, Radio box
public class Ex15 {
public static void main(String[] args) {
Frame f = new Frame();
f.setLayout(new FlowLayout());
f.setSize(500, 400);
f.setLocation(2560/2-250, 1600/2-200);
f.setVisible(true);
CheckboxGroup group = new CheckboxGroup();
Checkbox box1 = new Checkbox("check1", true, group);
box1.setFont(new Font("", 0, 30));
Checkbox box2 = new Checkbox("check2", false, group);
box2.setFont(new Font("", 0, 30));
Checkbox box3 = new Checkbox("check3", false, group);
box3.setFont(new Font("", 0, 30));
f.add(box1);
f.add(box2);
f.add(box3);
}
}
Choice, List, 그리고 Label
public class Ex16 {
public static void main(String[] args) {
Frame f = new Frame();
f.setLayout(new FlowLayout());
f.setSize(500, 400);
f.setLocation(2560/2-250, 1600/2-200);
f.setVisible(true);
Choice cho1 = new Choice();
cho1.addItem("item1");
cho1.addItem("item2");
cho1.addItem("item3");
cho1.addItem("item4");
cho1.select(1);
cho1.setFont(new Font("", 0, 15));
f.add(cho1);
java.awt.List list = new List(5);
// java.awt.List list = new List(5, true); // 다중선택
list.add("item1");
list.add("item2");
list.add("item3");
list.add("item4");
list.add("item5");
f.add(list);
Label la = new Label();
la.setText("Label");
f.add(la);
JLabel jla = new JLabel();
jla.setIcon(new ImageIcon("logo.jpg"));
f.add(jla);
}
}
'100일 챌린지 > 빅데이터기반 인공지능 융합 서비스 개발자' 카테고리의 다른 글
Day 14 - 예외 처리, I/O (0) | 2024.08.08 |
---|---|
Day 13 - UI 구현하기 (0) | 2024.08.07 |
Day 11 - generic, 순서가 없는 자료구조(Enumeration),그리고 Map (0) | 2024.08.05 |
Day10 - 동적할당, 자료 구조, List와 Set (0) | 2024.08.02 |
Day09 - Wrapper 클래스 (0) | 2024.08.01 |