React
React is the library for web and native user interfaces. Build user interfaces out of individual pieces called components written in JavaScript. React is designed to let you seamlessly combine components written by independent people, teams, and organizati
react.dev
단축키 사용하기
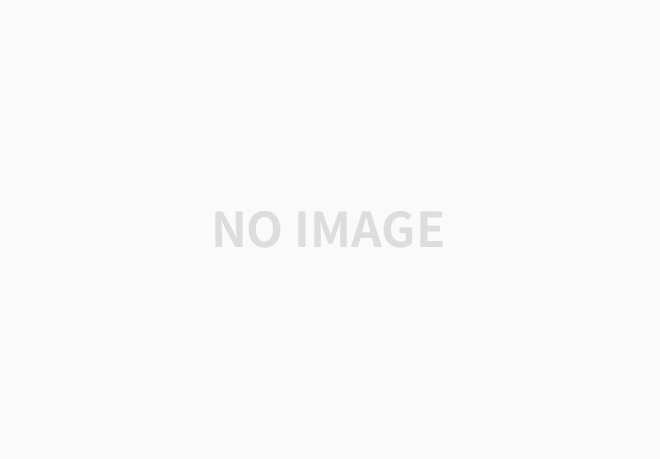
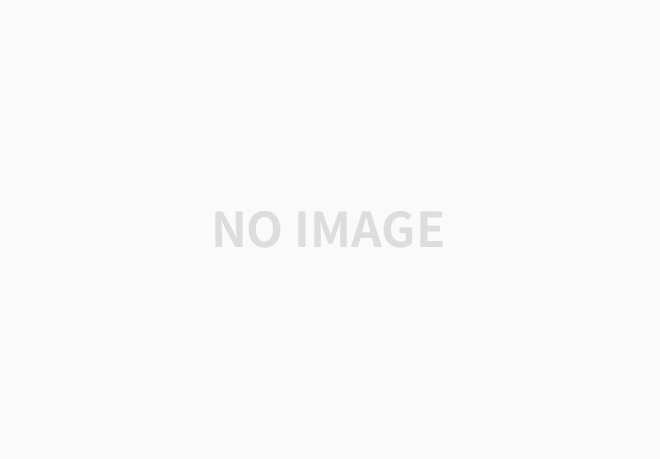
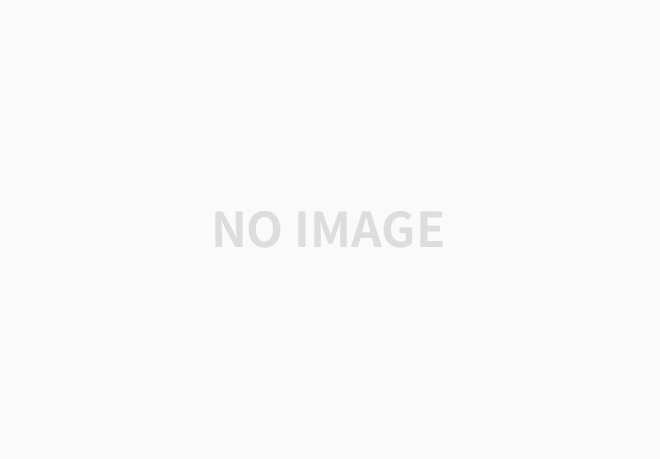
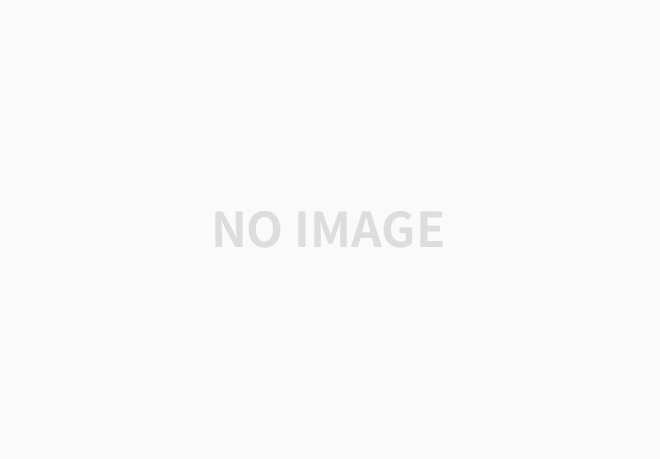
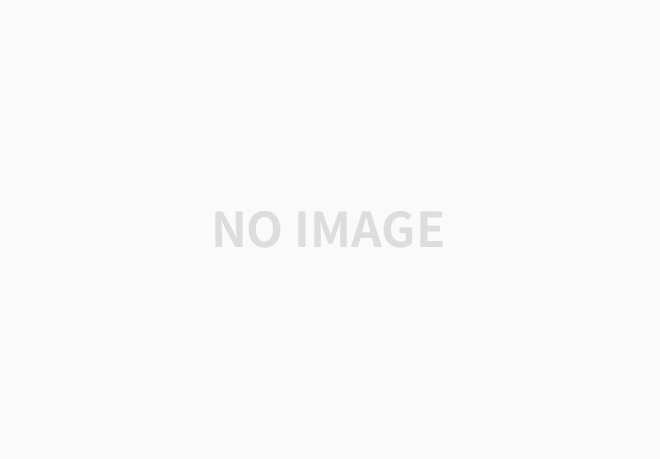
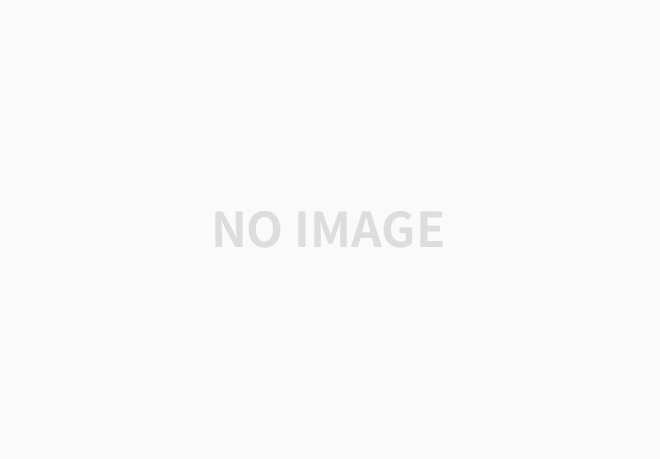
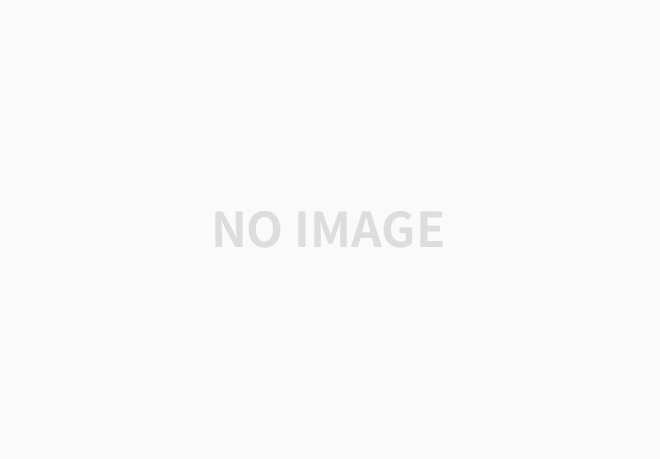
프로젝트 만들기
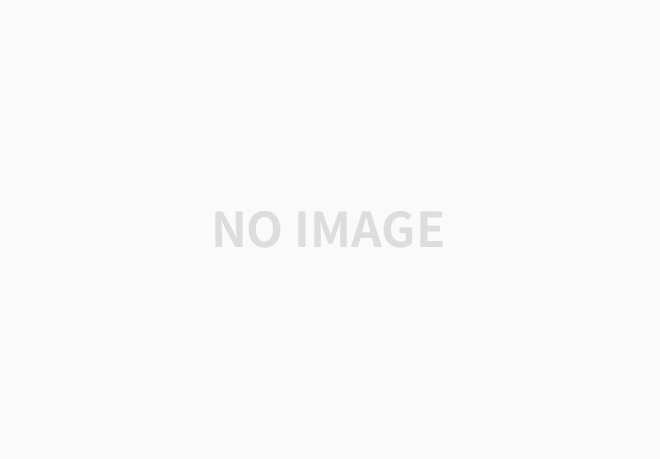
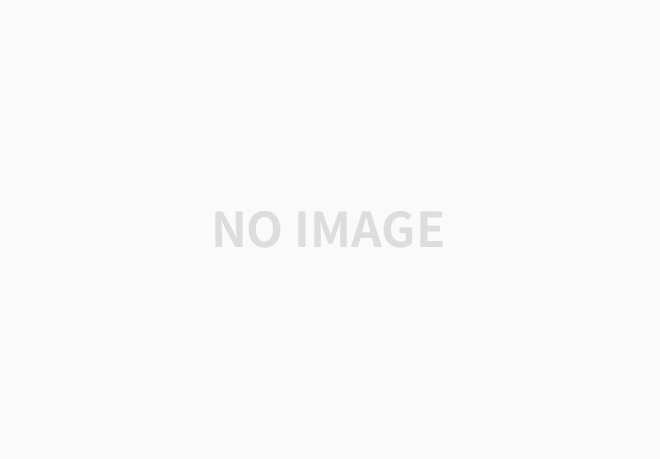
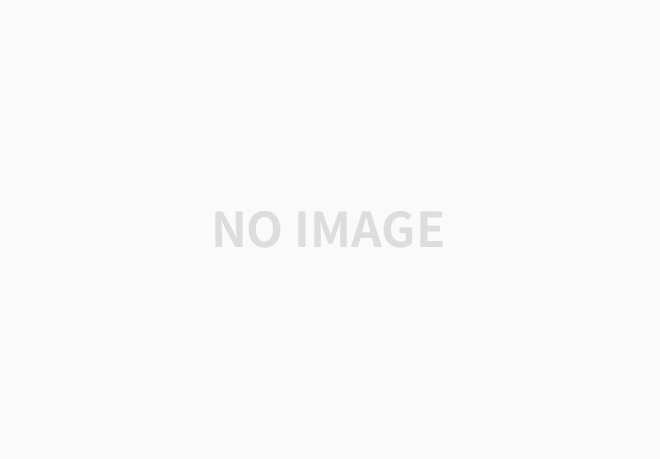
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const hr=React.createElement('hr');
const h1=React.createElement('h1',{},'MSG');
const a=React.createElement('a',{href:'http://google.com'},'link');
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(a);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
로 시작
jsx
https://ko.legacy.reactjs.org/docs/introducing-jsx.html
JSX 소개 – React
A JavaScript library for building user interfaces
ko.legacy.reactjs.org
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const hr=React.createElement('hr');
const h1=React.createElement('h1',{},'MSG');
const a=React.createElement('a',{href:'http://google.com'},'link');
const h1_test=<h1>test page</h1>;
const btn=<button onclick="console.log('test');">btn</button>
// const xml=<div><h1>test</h1><p>text</p></div>;
// const xml=<React.Fragment><h1>test</h1><p>text</p></React.Fragment>;
const xml=<>{h1_test}<p>text</p></>;
class Car extends React.Component {
constructor(){
super();
this.state={su:1111, color:"blue"}
}
changeColor=()=>{
this.setState({color:"orange"});
}
// 컴포넌트가 수정됐을때
componentDidUpdate(){
console.log('바뀜');
}
// 컴포넌트가 없어졌을때
componentDidMount(){
}
render() {
return (<><h2>Class {this.state.color} Car "{this.props.name}": {this.state.su}</h2>
<button type="button" onClick={this.changeColor}>changeColor</button>
</>);
}
}
function Car2(){
return <h2>Function Car2</h2>;
}
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<Car name="myCar"/>);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
Hook(함수형) 사용하기
function Car(props){
const [color,setColor]=useState(props.color);
const [info,setInfo]=useState({model:'모닝',company:'현대'});
useEffect(()=>{
console.log('call...');
},[]);
const changeColor=()=>{
setColor('blue');
};
const changeModel=()=>{
setInfo({...info,model:'그랜저'});
};
return (<>
<h1>내 차 {props.name}</h1>
<p>{info.company}에서 출시한 {info.model}</p>
<p>색은 {color}</p>
<button type="button" onClick={changeColor}>changeColor</button>
<button type="button" onClick={changeModel}>changeModel</button>
</>);
}
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<Car name="붕붕이" color="red"/>);
import './App.css';
import logo from './logo192.png';
function App02(){
return <h1>ex02 page</h1>;
}
function App03(){
return <h1>ex03 page</h1>;
}
// function App04(){
// return <h1>ex04 page</h1>;
// }
// export const App01=App02;
// export const App00=App03;
export {App02,App03};
export default function (){
return <><h1>ex04 page</h1><img src={logo}/></>;
};
import React, { useEffect, useState } from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import App04,{App01,App00} from './App02';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App04/>);
Event
import React from 'react'
function App05() {
const btnClick=e=>{
console.log('클릭',e);
}
return (
<>
<h2>react event</h2>
<button onClick={
btnClick
// ()=>{
// console.log('click');
// }
}>click</button>
<button onClick={(e)=>{btnClick();}}>click</button>
</>
)
}
export default App05
Form
import React, { useState } from 'react'
function App06({msg}){
return <div id='target'>{msg}</div>;
}
function App05() {
let [msg,setMsg]=useState('초기값');
const btnClick=e=>{
console.log('클릭',e);
};
const formSubmit=e=>{
e.preventDefault();
console.log(msg);
};
return (
<>
<h2>react event</h2>
<form onSubmit={formSubmit}>
<input onChange={e=>{setMsg(e.target.value);}} value={msg}/>
<button type='submit'>전달</button>
</form>
<button onClick={
btnClick
// ()=>{
// console.log('click');
// }
}>click</button>
<button onClick={(e)=>{btnClick();}}>click</button>
<App06 msg={msg}/>
</>
)
}
export default App05
Input
import React, { useState } from 'react';
function App07() {
const [input1,setInput1]=useState('');
const [input2,setInput2]=useState('');
return (
<div>
<input value={input1} onChange={e=>{setInput1(e.target.value);}}/>
<input value={input2} onChange={e=>{setInput2(e.target.value);}}/>
</div>
);
}
export default App07;
import React, { useState } from 'react';
function App07() {
// const [input1,setInput1]=useState('');
// const [input2,setInput2]=useState('');
const [inputs,setInputs]=useState({input1:'',input2:''});
const inputFunc=e=>{
if(e.target.name=='deptno')
setInputs({...inputs,input1:e.target.value});
if(e.target.name=='dname')
setInputs({...inputs,input2:e.target.value});
};
return (
<div>
<input value={inputs.input1} name='deptno' onChange={inputFunc}/>
<input value={inputs.input2} name='dname' onChange={inputFunc}/>
</div>
);
}
export default App07;
'100일 챌린지 > 빅데이터기반 인공지능 융합 서비스 개발자' 카테고리의 다른 글
Day 78 - React 프로그램 배포하기 (3) | 2024.11.19 |
---|---|
Day 78 - React로 CRUD 프로그램 만들기 (1) dummy json 사용 (0) | 2024.11.19 |
Day 77 - Nodejs express로 CRUD 프로그램 만들기 (0) | 2024.11.18 |
Day 75 - interface, lamda식, stream (0) | 2024.11.14 |
Day 74 - JavaScript로 Web Server 만들기 (0) | 2024.11.13 |