목차
프로젝트 만들기
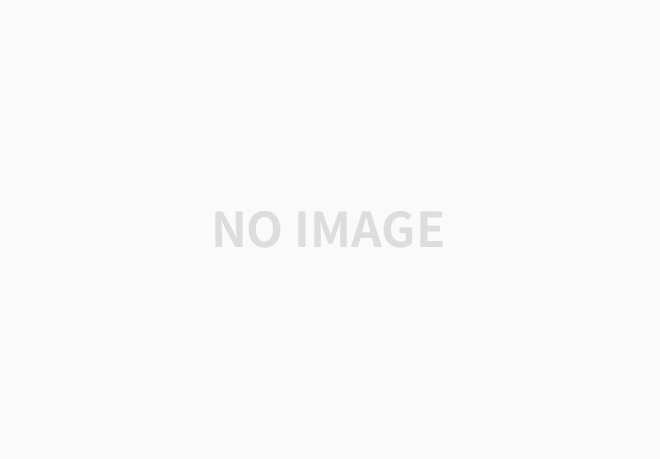
react router dom 사용하기
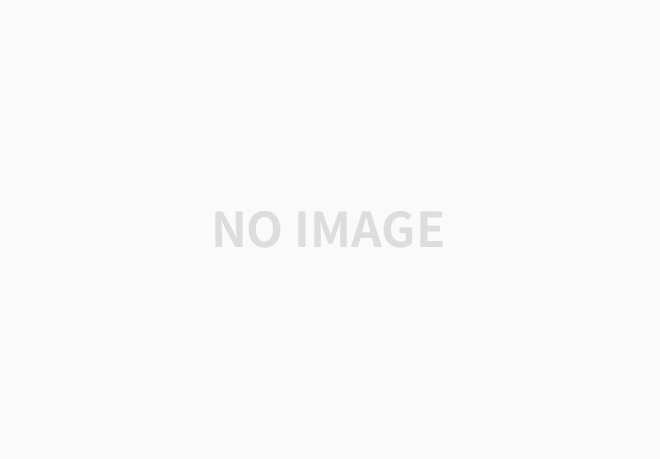
https://www.w3schools.com/react/react_router.asp
W3Schools.com
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
https://reactrouter.com/en/main/start/overview
Feature Overview | React Router
reactrouter.com
실행시키기
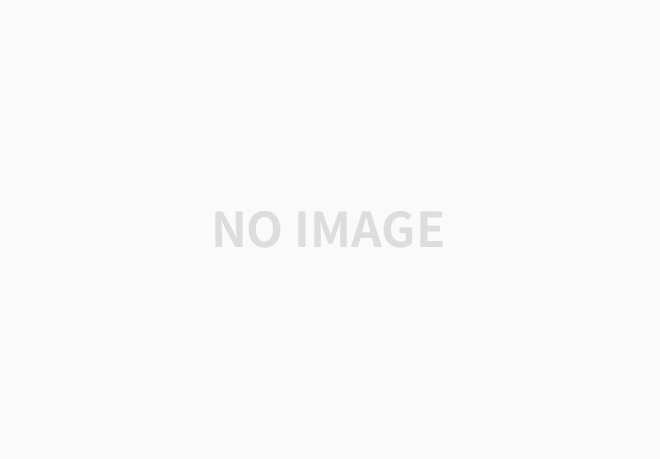
더보기
페이지 이동시키기(reate router없이)
import { useEffect, useState } from 'react';
import logo from './logo.svg';
// import './App.css';
import Home from './pages/Home';
import Intro from './pages/Intro';
import Dept from './pages/Dept';
function App() {
const[url,setUrl]=useState();
const[comm,setComm]=useState('/');
useEffect(()=>{
setUrl(window.location.href.replace('http://localhost:3000/',''));
},[]);
useEffect(()=>{
if(window.location.href.replace('http://localhost:3000/','')==''){
setComm(<Home/>);
}else if(window.location.href.replace('http://localhost:3000/','')=='#'){
setComm(<Home/>);
}else if(window.location.href.replace('http://localhost:3000/','')=='#intro'){
setComm(<Intro/>);
}else if(window.location.href.replace('http://localhost:3000/','')=='#dept'){
setComm(<Dept/>);
}
},[url]);
const linkClick=e=>{
setUrl(window.location.href.replace('http://localhost:3000/',''))
};
return (
<div className="App">
<nav>
<ul>
<li><a href='/#' onClick={linkClick}>home</a></li>
<li><a href='/#intro' onClick={linkClick}>intro</a></li>
<li><a href='/#dept' onClick={linkClick}>dept</a></li>
</ul>
</nav>
{comm}
</div>
);
}
export default App;
Child component 사용하기
import React from 'react'
function Ex03() {
return (
<div>inner component</div>
)
}
export default Ex03
import React, { useEffect } from 'react'
import Ex03 from './Ex03';
function Ex01(props) {
useEffect(()=>{
console.log(props);
});
return (
<>
{props.children}
<div>su:{props.su}</div>
<div>name:{props.name}</div>
</>
)
}
export default Ex01
import React from 'react'
import Ex01 from './Ex01'
import Ex03 from './Ex03'
function Ex02() {
return (
<div>
<h2>outter component</h2>
<Ex01 su={1111} name={'test'}><Ex03/></Ex01>
</div>
)
}
export default Ex02
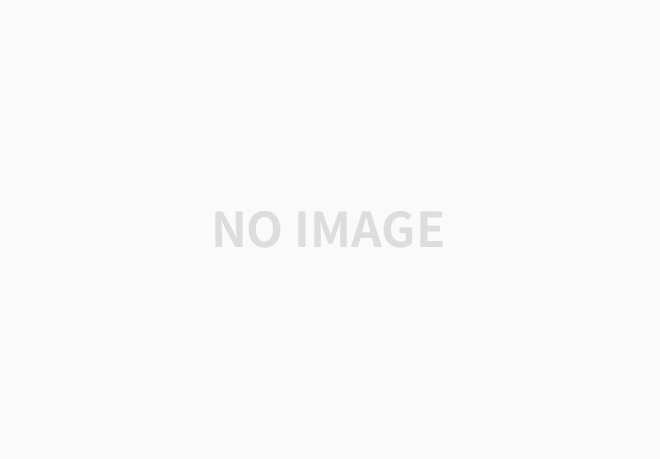
React Router 사용하기
index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import Home from './pages/Home';
import Ex02 from './Ex02';
import { BrowserRouter, Route, Router, Routes } from 'react-router-dom';
import Intro from './pages/Intro';
import Depts from './pages/Depts';
import Dept from './pages/Dept';
import AddDept from './pages/AddDept';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<BrowserRouter>
<Routes>
<Route>
<Route path='/' element={<Home/>}/>
<Route path='/intro' element={<Intro/>}/>
<Route path='/dept' element={<Depts/>}/>
<Route path='/dept/:id' element={<Dept/>}/>
<Route path='/dept/add' element={<AddDept/>}/>
</Route>
</Routes>
</BrowserRouter>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
Home.js
import React, { useEffect } from 'react'
import { Link } from 'react-router-dom'
function Home() {
useEffect(()=>{
console.log('home');
},[]);
const st_li={float:'left',width:98,marginLeft:1,marginRight:1};
const st_Link={
display:'block',height:35,backgroundColor:'gray',
textAlign:'center',color:'white',textDecoration:'none'};
return (
<>
<nav style={{clear:'left',height:35,borderBottom:'1px solid gray'}}>
<ul style={{listStyle:'none',padding:0,width:300,margin:'auto'}}>
<li style={st_li}><Link to={'/'} style={st_Link}>Home</Link></li>
<li style={st_li}><Link to={'/intro'} style={st_Link}>Intro</Link></li>
<li style={st_li}><Link to={'/dept/'} style={st_Link}>Dept</Link></li>
</ul>
</nav>
<h2>Index page</h2>
</>
)
}
export default Home
Intro.js
import React, { useEffect } from 'react'
import { Link } from 'react-router-dom'
function Intro() {
useEffect(()=>{
console.log('intro');
},[]);
const st_li={float:'left',width:98,marginLeft:1,marginRight:1};
const st_Link={
display:'block',height:35,backgroundColor:'gray',
textAlign:'center',color:'white',textDecoration:'none'};
return (
<>
<nav style={{clear:'left',height:35,borderBottom:'1px solid gray'}}>
<ul style={{listStyle:'none',padding:0,width:300,margin:'auto'}}>
<li style={st_li}><Link to={'/'} style={st_Link}>Home</Link></li>
<li style={st_li}><Link to={'/intro'} style={st_Link}>Intro</Link></li>
<li style={st_li}><Link to={'/dept/'} style={st_Link}>Dept</Link></li>
</ul>
</nav>
<h2>Intro page</h2>
<img src='https://www.inje.ac.kr/kor/assets/images/sub/gimhae-campus-1-241008.jpg'/>
</>
)
}
export default Intro
Depts,js
import React, { useEffect, useState } from 'react'
import { Link } from 'react-router-dom'
function Dept() {
const[arr,setArr]=useState(['item1','item2']);
useEffect(()=>{
fetch('https://dummyjson.com/products')
.then(e=>e.json())
.then(data=>{
setArr(data.products);
});
},[]);
const styleLi={float:'left',width:98,marginLeft:1,marginRight:1};
const styleLink={
display:'block',height:35,backgroundColor:'gray',
textAlign:'center',color:'white',textDecoration:'none'};
const st_lineTop={borderTop:'1px solid gray'};
const st_lineBtm={borderBottom:'1px solid gray'};
const st_Link={textDecoration:'none',color:'gray'};
return (
<>
<nav style={{clear:'left',height:35,borderBottom:'1px solid gray'}}>
<ul style={{listStyle:'none',padding:0,width:300,margin:'auto'}}>
<li style={styleLi}><Link to={'/'} style={styleLink}>Home</Link></li>
<li style={styleLi}><Link to={'/intro'} style={styleLink}>Intro</Link></li>
<li style={styleLi}><Link to={'/dept/'} style={styleLink}>Dept</Link></li>
</ul>
</nav>
<h2>List page</h2>
<p><Link to={'/dept/add'}>입력</Link></p>
<table style={{width:'100%'}}>
<thead>
<tr>
<th style={st_lineBtm}>id</th>
<th style={st_lineBtm}>category</th>
<th style={st_lineBtm}>title</th>
<th style={st_lineBtm}>price</th>
</tr>
</thead>
<tbody>
{arr.map((ele,idx)=>{
return(
<tr>
<td style={st_lineTop}><Link style={st_Link} to={'/dept/'+ele.id}>{ele.id}</Link></td>
<td style={st_lineTop}><Link style={st_Link} to={'/dept/'+ele.id}>{ele.category}</Link></td>
<td style={st_lineTop}><Link style={st_Link} to={'/dept/'+ele.id}>{ele.title}</Link></td>
<td style={st_lineTop}><Link style={st_Link} to={'/dept/'+ele.id}>{ele.price}</Link></td>
</tr>
);
})}
</tbody>
</table>
</>
)
}
export default Dept
Dept.js
import React, { useEffect, useState } from 'react'
import { Link, useParams } from 'react-router-dom'
function Dept() {
const {id}=useParams();
const [bean,setBean]=useState({});
useEffect(()=>{
fetch('https://dummyjson.com/products/'+id)
.then(e=>e.json())
.then(data=>{
setBean(data);
});
},[]);
const st_li={float:'left',width:98,marginLeft:1,marginRight:1};
const st_Link={
display:'block',height:35,backgroundColor:'gray',
textAlign:'center',color:'white',textDecoration:'none'};
return (
<>
<nav style={{clear:'left',height:35,borderBottom:'1px solid gray'}}>
<ul style={{listStyle:'none',padding:0,width:300,margin:'auto'}}>
<li style={st_li}><Link to={'/'} style={st_Link}>Home</Link></li>
<li style={st_li}><Link to={'/intro'} style={st_Link}>Intro</Link></li>
<li style={st_li}><Link to={'/dept/'} style={st_Link}>Dept</Link></li>
</ul>
</nav>
<h2>Detail Page</h2>
<form>
<div><input name='id' value={bean.id} readonly/></div>
<div><input name='category' value={bean.category} readonly/></div>
<div><input name='title' value={bean.title} readonly/></div>
<div><input name='price' value={bean.price} readonly/></div>
</form>
</>
)
}
export default Dept
AddDept.js
import React from 'react'
import { Link, useNavigate } from 'react-router-dom';
function AddDept() {
const navigate=useNavigate();
const formSubmit=e=>{
e.preventDefault();
console.log(e.target.category.value,e.target.title.value,e.target.price.value);
fetch('https://dummyjson.com/products/add', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({
category: e.target.category.value,
title: e.target.title.value,
price: e.target.price.value,
})
})
.then(e=>e.json())
.then(data=>{
navigate('/dept/');
});
};
const styleLi={float:'left',width:98,marginLeft:1,marginRight:1};
const styleLink={
display:'block',height:35,backgroundColor:'gray',
textAlign:'center',color:'white',textDecoration:'none'};
const st_display={width:'80%',marginLeft:'10%'};
return (
<>
<nav style={{clear:'left',height:35,borderBottom:'1px solid gray'}}>
<ul style={{listStyle:'none',padding:0,width:300,margin:'auto'}}>
<li style={styleLi}><Link to={'/'} style={styleLink}>Home</Link></li>
<li style={styleLi}><Link to={'/intro'} style={styleLink}>Intro</Link></li>
<li style={styleLi}><Link to={'/dept/'} style={styleLink}>Dept</Link></li>
</ul>
</nav>
<h2>Add Page</h2>
<form style={st_display} onSubmit={formSubmit}>
<div><input style={st_display} name='category' placeholder='category'/></div>
<div><input style={st_display} name='title' placeholder='title'/></div>
<div><input style={st_display} name='price' placeholder='price'/></div>
<div>
<button style={st_display}>입력</button>
</div>
</form>
</>
)
}
export default AddDept
'100일 챌린지 > 빅데이터기반 인공지능 융합 서비스 개발자' 카테고리의 다른 글
Day 78 - React로 CRUD 프로그램 만들기 (2) spring에서 데이터셋 활용하기 [h2] (1) | 2024.11.19 |
---|---|
Day 78 - React 프로그램 배포하기 (3) | 2024.11.19 |
Day 77 - React 사용하기, 문법(1) (0) | 2024.11.18 |
Day 77 - Nodejs express로 CRUD 프로그램 만들기 (0) | 2024.11.18 |
Day 75 - interface, lamda식, stream (0) | 2024.11.14 |